Section Headers
The Section Headers follow immediately after the Optional Header, and contain information about the various sections of the PE file.
PE Section Table
Each section has a corresponding Section Header. Taken together, the Section Headers comprise the Section Table, with each row of the Section Table containing a Section Header.
The following image shows the Section Table of a PE file, with each row corresponding to a Section Header:
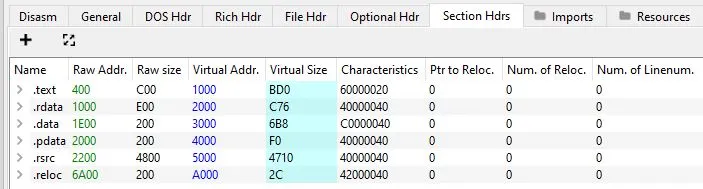
Note that each Section Header corresponds to a section in the PE: .text, .rdata, .data. .pdata, .rsrc, .reloc.
Section Header Struct
Each Section header has the following structure:
typedef struct _IMAGE_SECTION_HEADER {
BYTE Name[IMAGE_SIZEOF_SHORT_NAME];
union {
DWORD PhysicalAddress;
DWORD VirtualSize;
} Misc;
DWORD VirtualAddress;
DWORD SizeOfRawData;
DWORD PointerToRawData;
DWORD PointerToRelocations;
DWORD PointerToLinenumbers;
WORD NumberOfRelocations;
WORD NumberOfLinenumbers;
DWORD Characteristics;
} IMAGE_SECTION_HEADER, *PIMAGE_SECTION_HEADER;
Section Header Elements
Name
The first member of the Section Header is the name of the section. It’s actually a byte array with a size of IMAGE_SIZEOF_SHORT_NAME, which is set to 8, meaning that the maximum number of allowed characters is 8.
PhysicalAddress/VirualSize
The size of the section when it’s in memory.
VirtualAddress
The offset to the start of the section in memory.
SizeOfRawData
The size of the section on disk. Must be a multiple of the FileAlignment member of the Optional Header, i.e. IMAGE_OPTIONAL_HEADER.FileAlignment.
PointerToRawData
File pointer to the first page of the section.
PointerToRelocations
File pointer to the beginning of the relocation entries for the section.
PointerToLinenumbers
File pointer to the beginning of the line-number entries for the section
NumberOfRelocations
The number of relocation entries. For executables, this is set to zero (0).
NumberOfLinenumbers
Number of line-number entries for the section. This is no longer used and is set to zero (0).
Characteristics
A DWORD that uses flags to signal various characteristics of the PE. For reference, the following table shows the defined flags:
Flag | Name | Meaning |
---|---|---|
0x00000000 | Reserved. | |
0x00000001 | Reserved. | |
0x00000002 | Reserved. | |
0x00000004 | Reserved. | |
0x00000008 | IMAGE_SCN_TYPE_NO_PAD | The section should not be padded to the next boundary. This flag is obsolete and was replaced by IMAGE_SCN_ALIGN_1BYTES. |
0x00000010 | Reserved. | |
0x00000020 | IMAGE_SCN_CNT_CODE | The section contains executable code. |
0x00000040 | IMAGE_SCN_CNT_INITIALIZED_DATA | The section contains initialized data. |
0x00000080 | IMAGE_SCN_CNT_UNINITIALIZED_DATA | The section contains uninitialized data. |
0x00000100 | IMAGE_SCN_LNK_OTHER | Reserved. |
0x00000200 | IMAGE_SCN_LNK_INFO | The section contains comments or other information. Valid only for object files. |
0x00000400 | Reserved. | |
0x00000800 | IMAGE_SCN_LNK_REMOVE | The section will not become part of the image. Valid only for object files. |
0x00001000 | IMAGE_SCN_LNK_COMDAT | The section contains COMDAT data. Valid only for object files. |
0x00002000 | Reserved. | |
0x00004000 | IMAGE_SCN_NO_DEFER_SPEC_EXC | Reset speculative exceptions handling bits in the TLB entries for this section. |
0x00008000 | IMAGE_SCN_GPREL | The section contains data referenced through the global pointer. |
0x00010000 | Reserved. | |
0x00020000 | IMAGE_SCN_MEM_PURGEABLE | Reserved. |
0x00040000 | IMAGE_SCN_MEM_LOCKED | Reserved. |
0x00080000 | IMAGE_SCN_MEM_PRELOAD | Reserved. |
0x00100000 | IMAGE_SCN_ALIGN_1BYTES | Align data on a 1-byte boundary. Valid only for object files. |
0x00200000 | IMAGE_SCN_ALIGN_2BYTES | Align data on a 2-byte boundary. Valid only for object files. |
0x00300000 | IMAGE_SCN_ALIGN_4BYTES | Align data on a 4-byte boundary. Valid only for object files. |
0x00400000 | IMAGE_SCN_ALIGN_8BYTES | Align data on a 8-byte boundary. Valid only for object files. |
0x00500000 | IMAGE_SCN_ALIGN_16BYTES | Align data on a 16-byte boundary. Valid only for object files. |
0x00600000 | IMAGE_SCN_ALIGN_32BYTES | Align data on a 32-byte boundary. Valid only for object files. |
0x00700000 | IMAGE_SCN_ALIGN_64BYTES | Align data on a 64-byte boundary. Valid only for object files. |
0x00800000 | IMAGE_SCN_ALIGN_128BYTES | Align data on a 128-byte boundary. Valid only for object files. |
0x00900000 | IMAGE_SCN_ALIGN_256BYTES | Align data on a 256-byte boundary. Valid only for object files. |
0x00A00000 | IMAGE_SCN_ALIGN_512BYTES | Align data on a 512-byte boundary. Valid only for object files. |
0x00B00000 | IMAGE_SCN_ALIGN_1024BYTES | Align data on a 1024-byte boundary. Valid only for object files. |
0x00C00000 | IMAGE_SCN_ALIGN_2048BYTES | Align data on a 2048-byte boundary. Valid only for object files. |
0x00D00000 | IMAGE_SCN_ALIGN_4096BYTES | Align data on a 4096-byte boundary. Valid only for object files. |
0x00E00000 | IMAGE_SCN_ALIGN_8192BYTES | Align data on a 8192-byte boundary. Valid only for object files. |
0x01000000 | IMAGE_SCN_LNK_NRELOC_OVFL | The section contains extended relocations. See the Microsoft documentation for more information. |
0x02000000 | IMAGE_SCN_MEM_DISCARDABLE | The section can be discarded as needed. |
0x04000000 | IMAGE_SCN_MEM_NOT_CACHED | The section cannot be cached. |
0x08000000 | IMAGE_SCN_MEM_NOT_PAGED | The section cannot be paged. |
0x10000000 | IMAGE_SCN_MEM_SHARED | The section can be shared in memory. |
0x20000000 | IMAGE_SCN_MEM_EXECUTE | The section can be executed as code. |
0x40000000 | IMAGE_SCN_MEM_READ | The section can be read. |
0x80000000 | IMAGE_SCN_MEM_WRITE | The section can be written to. |